Unlocking Efficiency: Angular and React Performance Best Practices
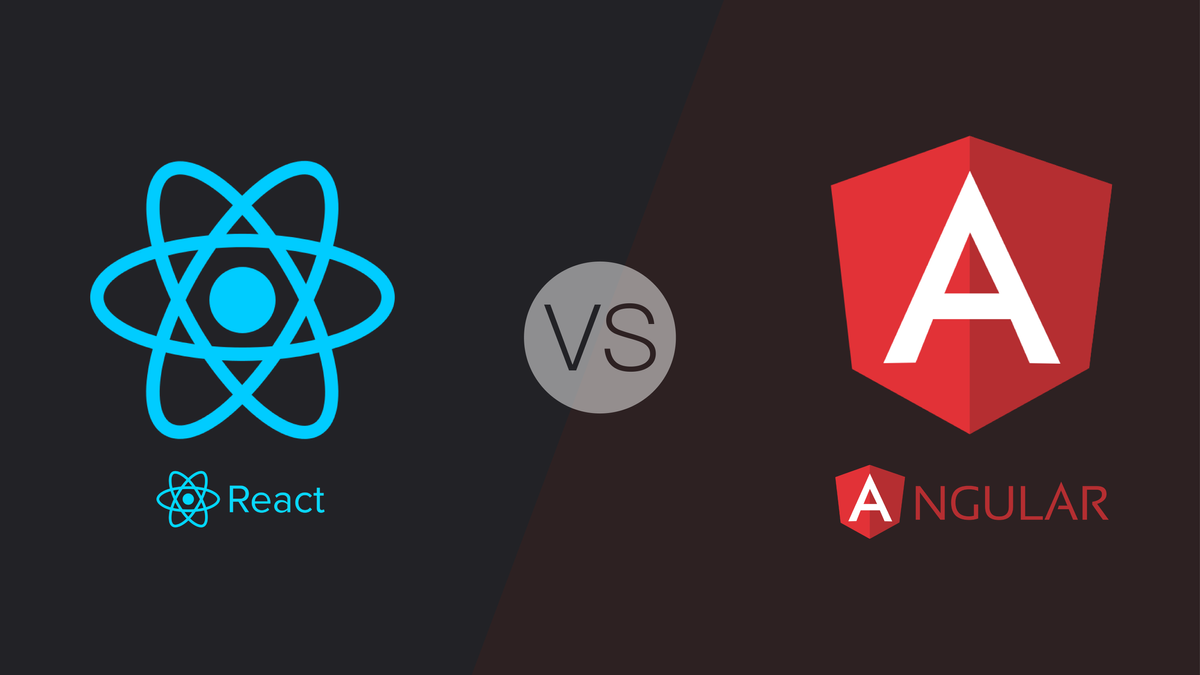
In the realm of web development, crafting high-performing applications is not just a goal; it's a necessity. Users expect swift interactions and seamless experiences, and any lag or delay can significantly impact user satisfaction and retention. In this article, we delve into the optimization strategies and best practices tailored for Angular and React applications. By implementing these techniques, developers can enhance the performance of their applications and deliver exceptional user experiences.
Understanding the Landscape:
Both Angular and React are powerful frameworks for building dynamic and interactive web applications. However, without proper optimization, even the most well-designed applications can suffer from performance bottlenecks. To overcome these challenges, developers must employ a combination of architectural principles and cutting-edge techniques.
React Optimization Strategies:
React.memo() for Memoization:
React.memo() is a Higher Order Component (HOC) that memoizes the rendering of functional components, preventing unnecessary re-renders when the component's props remain unchanged. By wrapping components with React.memo(), developers can optimize rendering performance and reduce the workload on the Virtual DOM reconciliation process.
const MemoizedComponent = React.memo((props) => {
return <div>{props.text}</div>;
});
useMemo() for Memoization in Functional Components:
Similar to React.memo(), the useMemo() hook memoizes the result of expensive computations in functional components. By memoizing values between renders, useMemo() optimizes performance by avoiding redundant calculations, especially in scenarios where complex data processing is involved.
const memoizedValue = useMemo(() => expensiveCalculation(dependencies), [dependencies]);
React.PureComponent for Class Components:
React.PureComponent is a base class for class components that implements a shallow comparison of props and state to determine if a component should update. By default, React components re-render whenever their parent component re-renders, but PureComponent optimizes this process by preventing unnecessary updates when props and state remain unchanged.
class MyComponent extends React.PureComponent {
render() {
return <div>{this.props.text}</div>;
}
}
Using Functional Components with Hooks:
Functional components with Hooks have gained popularity in React development due to their simplicity and performance benefits. Hooks like useState, useEffect, and useCallback enable developers to manage state, perform side effects, and optimize re-renders more efficiently than traditional class components.
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
};
Conclusion:
By combining Angular and React optimization strategies, developers can propel their web applications to new heights of performance and user satisfaction. Whether you're building a complex enterprise-grade application or a sleek single-page interface, adopting these best practices ensures your applications are finely tuned for speed and efficiency. With Angular's AOT compilation, lazy loading, and change detection optimization, alongside React's memoization, PureComponent, and Hooks, developers have a comprehensive toolkit at their disposal for crafting blazing-fast web experiences. Embrace these best practices, iterate on performance, and elevate your applications to the next level of excellence.
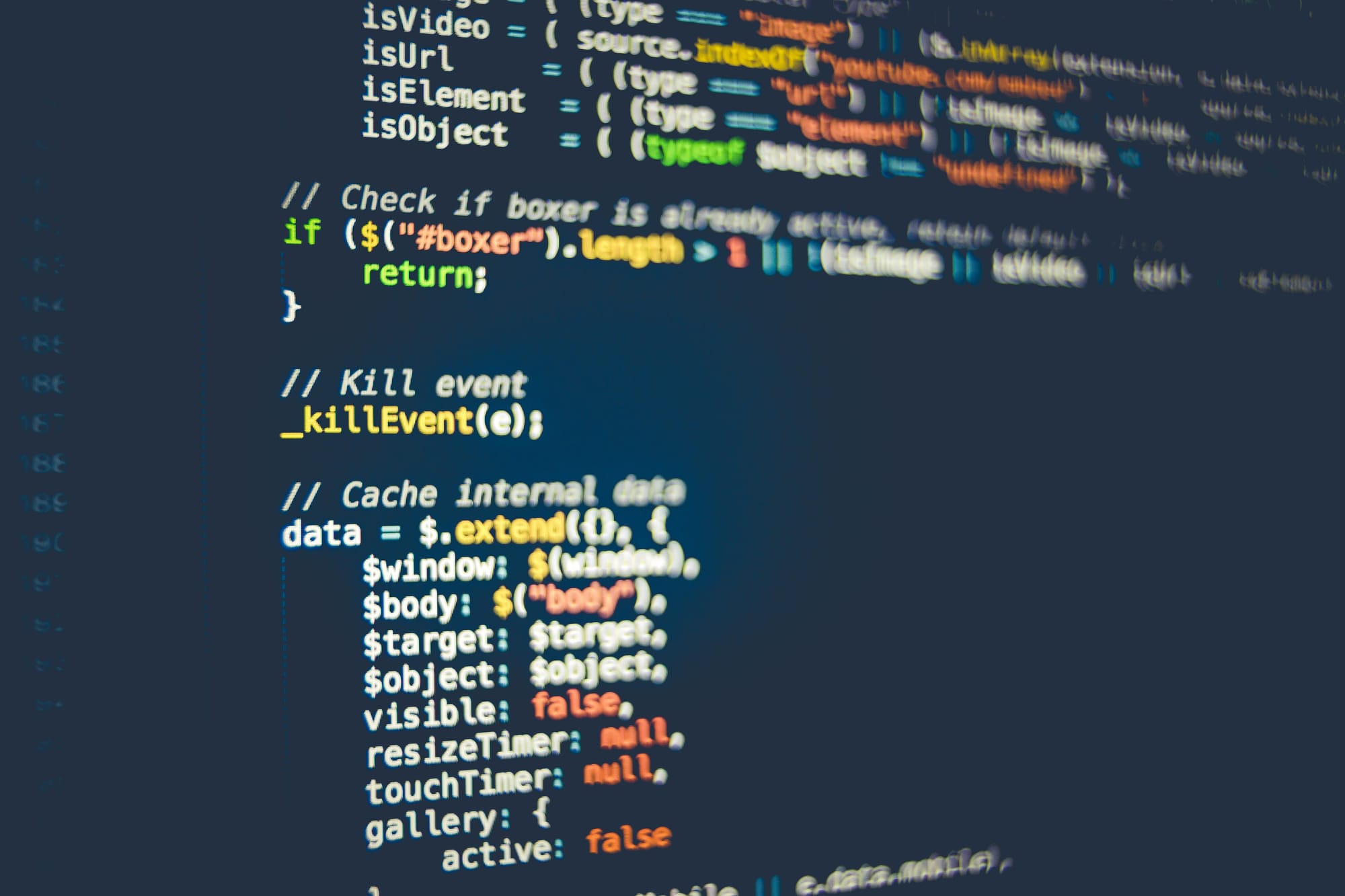
Angular Optimization Strategies:
Ahead-of-Time (AOT) Compilation:
Angular offers Ahead-of-Time (AOT) compilation as a default compilation option, unlike its Just-in-Time (JIT) counterpart. AOT compilation converts Angular HTML and TypeScript code into efficient JavaScript code during the build process. This eliminates the need for compilation in the browser, resulting in faster startup times and reduced bundle sizes.
ng build --prod --aot
Lazy Loading Modules:
Similar to React's code splitting, Angular supports lazy loading modules to defer the loading of certain parts of the application until they are needed. By splitting the application into smaller feature modules and lazy loading them on demand, developers can reduce initial load times and improve overall performance.
const routes: Routes = [
{ path: 'lazy', loadChildren: () => import('./lazy/lazy.module').then(m => m.LazyModule) }
];
Angular Universal for Server-Side Rendering (SSR):
Angular Universal enables developers to perform server-side rendering (SSR) of Angular applications. SSR delivers pre-rendered HTML to the client, significantly reducing time-to-content and improving perceived performance, especially for initial page loads. Additionally, SSR enhances search engine optimization (SEO) by ensuring content is readily accessible to web crawlers.
import { ngExpressEngine } from '@nguniversal/express-engine';
import { provideModuleMap } from '@nguniversal/module-map-ngfactory-loader';
app.engine('html', ngExpressEngine({
bootstrap: AppServerModuleNgFactory,
providers: [provideModuleMap(LAZY_MODULE_MAP)]
}));
Optimizing Change Detection:
Angular's change detection mechanism plays a crucial role in updating the application's view when data changes. However, inefficient change detection can lead to performance bottlenecks, especially in large applications. Developers can optimize change detection by leveraging techniques such as OnPush change detection strategy, immutable data structures, and using trackBy function in ngFor loops.
@Component({
selector: 'app-item',
templateUrl: './item.component.html',
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ItemComponent {
@Input() item: Item;
}
Conclusion:
In the dynamic landscape of web development, optimizing the performance of Angular and React applications is paramount for delivering exceptional user experiences. By incorporating Angular-specific techniques such as AOT compilation, lazy loading modules, Angular Universal for SSR, and optimizing change detection, developers can maximize the efficiency of their applications and stay ahead of the curve. Whether you're building a single-page application or a complex enterprise-grade solution, adopting these best practices ensures your Angular applications perform at their peak, setting the stage for unparalleled user satisfaction and engagement.