Micro-Frontends: Architecting Scalable UIs for the Modern Web
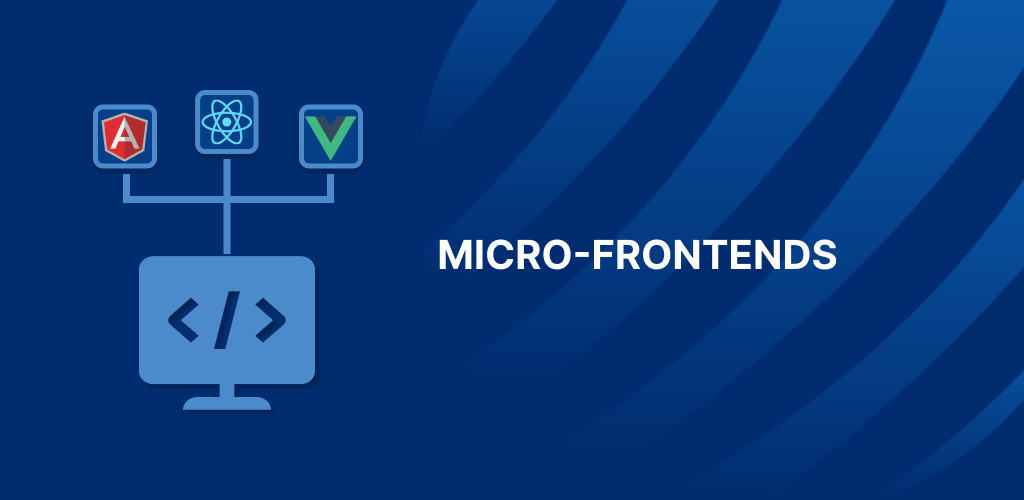
Building complex web applications often leads to monolithic front-ends – massive codebases that become unwieldy and difficult to maintain. Micro-frontends offer a solution, mirroring the success of microservices architectures on the backend. Let's dissect this approach and explore its potential.
Benefits of Micro-Frontends:
-
Independent Development and Ownership:
Teams can work on specific features (micro-frontends) using their preferred frameworks (Angular, React, Vue.js, etc.) without hindering others. -
Improved Maintainability:
Smaller codebases are easier to understand, test, and deploy. -
Scalability:
Teams can add new features or update existing ones independently, fostering a more agile development process. -
Fault Isolation:
Issues within a micro-frontend won't bring down the entire application.
Integrating Angular and React with Micro-Frontends:
Frameworks like Single-SPA and Module Federation enable seamless integration of different UI libraries within a micro-frontend architecture.
-
Single-SPA:
This popular framework facilitates dynamic loading and unloading of micro-frontends based on routes. Each micro-frontend can be built with Angular, React, or any other framework, promoting flexibility. -
Module Federation:
Introduced in Webpack 5, Module Federation allows micro-frontends to share dependencies and components dynamically. This is particularly useful for common UI elements like headers or navigation bars.
Best Practices for Micro-Frontend Development:
- Clear Communication and Ownership:
Define ownership boundaries for each micro-frontend and establish communication channels between teams. - Isolated Development Environments:
Encourage teams to use containerized environments or tools like Nx to manage dependencies and avoid conflicts. - Standardized Build and Deployment Processes:
Automate build and deployment pipelines for efficient micro-frontend updates. - Event-Driven Communication:
Utilize events to facilitate communication between micro-frontends without relying on a shared state.
Building a Sample Micro-Frontend Application (using Single-SPA):
Here's a simplified example showcasing how Single-SPA can integrate a React micro-frontend into an Angular shell application:
1 Setting up the Shell Application (Angular):
- Create a new Angular project using the Angular CLI.
- Define a route in your
app-routing.module.ts
for the micro-frontend:
const routes: Routes = [
{ path: 'products', component: ProductListComponent }, // Your Angular component
{ path: 'cart', loadChildren: () => import('cart/CartModule').then(m => m.CartModule) }, // Micro-frontend route (lazy loaded)
// ... other routes
];
2 Building the Micro-Frontend (React):
- Create a separate React project using tools like Create React App.
- Develop the UI components for the cart functionality.
- Utilize a library like
@single-spa/react
to integrate with Single-SPA.
import { registerApplication, start } from '@single-spa/react';
registerApplication(
'cart',
() => import('./cart'),
location => location.pathname.startsWith('/cart')
);
start();
This example demonstrates how a React micro-frontend can be loaded and displayed within an Angular shell application based on the URL.
Real-World Examples:
- Spotify utilizes micro-frontends to manage its complex web application, allowing different teams to focus on specific features like music playback or social elements.
- Netflix leverages micro-frontends to personalize the user experience by dynamically loading UI components based on user preferences.
By adopting micro-frontends, you can build robust and scalable UIs for modern web applications, fostering faster development cycles and empowering teams to work more efficiently. Remember, this is a conceptual overview. Consider exploring the frameworks mentioned above and referring to their documentation for in-depth implementation details.