Building Scalable and Maintainable UI Components in Angular and React
Learn how to create reusable and maintainable UI components in Angular and React, covering architecture, composition, data flow, directives, lifecycle methods, and testing.
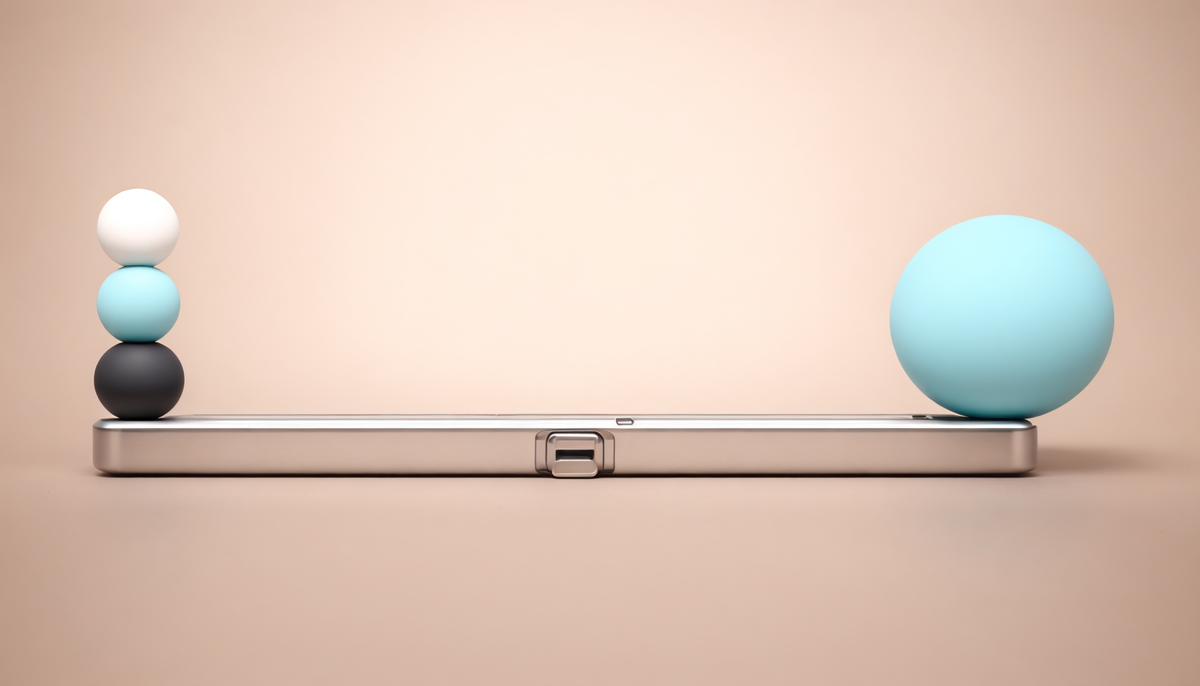
Introduction:
In the realm of modern web development, Angular and React stand as two pillars, offering robust frameworks for building dynamic user interfaces. However, crafting scalable and maintainable UI components within these frameworks requires thoughtful architecture and best practices. In this blog post, we'll delve into key strategies and techniques for creating reusable and maintainable UI components in Angular and React, covering component architecture, composition, props vs. inputs, directives, lifecycle methods, and testing.
Component Architecture:
Both Angular and React follow component-based architectures, wherein the UI is broken down into reusable and self-contained components. In Angular, components are the fundamental building blocks, encapsulating HTML templates, styles, and behavior. React, on the other hand, uses functional or class-based components to achieve similar encapsulation. When designing component architectures, prioritize the single responsibility principle, ensuring each component handles a specific aspect of the UI.
Component Composition:
One of the core principles of building maintainable UI components is composition over inheritance. Both Angular and React empower developers to compose complex UIs by combining smaller, reusable components. In Angular, this is achieved through component nesting and transclusion, allowing for hierarchical composition. React embraces composition through JSX syntax, enabling the creation of composite components with ease. By leveraging composition, developers can foster modularity and reusability in their UI codebase.
Props vs. Inputs:
In React, components communicate with each other through props, which are immutable properties passed from parent to child components. Similarly, Angular utilizes inputs to pass data from parent to child components. While the concept is similar, Angular inputs offer additional features such as type-checking and change detection mechanisms. When designing components, carefully consider the data flow and choose the appropriate mechanism for passing data between components.
Directives:
Angular introduces the concept of directives, which are markers on a DOM element that tell Angular to do something with that element. Directives can be structural, altering the DOM layout (e.g., *ngFor, *ngIf), or attribute-based, modifying the behavior or appearance of DOM elements (e.g., ngClass, ngStyle). React, on the other hand, relies on a more explicit approach, utilizing JavaScript functions and conditional rendering to achieve similar outcomes. When using directives in Angular or conditional rendering in React, maintain clarity and readability to enhance code maintainability.
Lifecycle Methods:
Understanding component lifecycle is crucial for building scalable and performant UI components. Both Angular and React provide lifecycle hooks that allow developers to perform actions at specific points in a component's lifecycle. In Angular, lifecycle hooks like ngOnInit, ngOnDestroy, and ngOnChanges facilitate initialization, cleanup, and reacting to input changes. React offers useEffect hook for handling side effects, useState for managing state within functional components, and lifecycle methods like componentDidMount for initialization. By leveraging lifecycle methods effectively, developers can optimize component performance and ensure proper resource management.
Component Testing:
Comprehensive testing is essential for validating the functionality and behavior of UI components. Angular provides robust testing utilities like TestBed and ComponentFixture for unit and integration testing of components. React, on the other hand, offers tools like Jest and React Testing Library for testing functional and class-based components. Regardless of the framework, focus on writing isolated unit tests for individual components and integration tests for component interactions. Embrace test-driven development (TDD) to foster code quality and maintainability throughout the development lifecycle.
Example Code (Angular):
// Example Angular component with inputs and lifecycle hooks
import { Component, Input, OnInit, OnDestroy } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent implements OnInit, OnDestroy {
@Input() data: any;
constructor() { }
ngOnInit(): void {
// Initialization logic
}
ngOnDestroy(): void {
// Cleanup logic
}
}
Example Code (React):
// Example React component with props and useEffect hook
import React, { useEffect } from 'react';
const ExampleComponent = ({ data }) => {
useEffect(() => {
// Initialization logic
return () => {
// Cleanup logic
};
}, []);
return (
<div>
{/* Component rendering */}
</div>
);
};
export default ExampleComponent;
Conclusion:
Building scalable and maintainable UI components in Angular and React requires a combination of architectural best practices, component composition, thoughtful data flow management, utilization of directives, understanding component lifecycles, and comprehensive testing strategies. By following these insights and adopting a pragmatic approach to component development, developers can create resilient, reusable, and easy-to-maintain UI components that form the foundation of exceptional user experiences in web applications.