Building a Real-Time Dashboard with WebSockets and Beyond
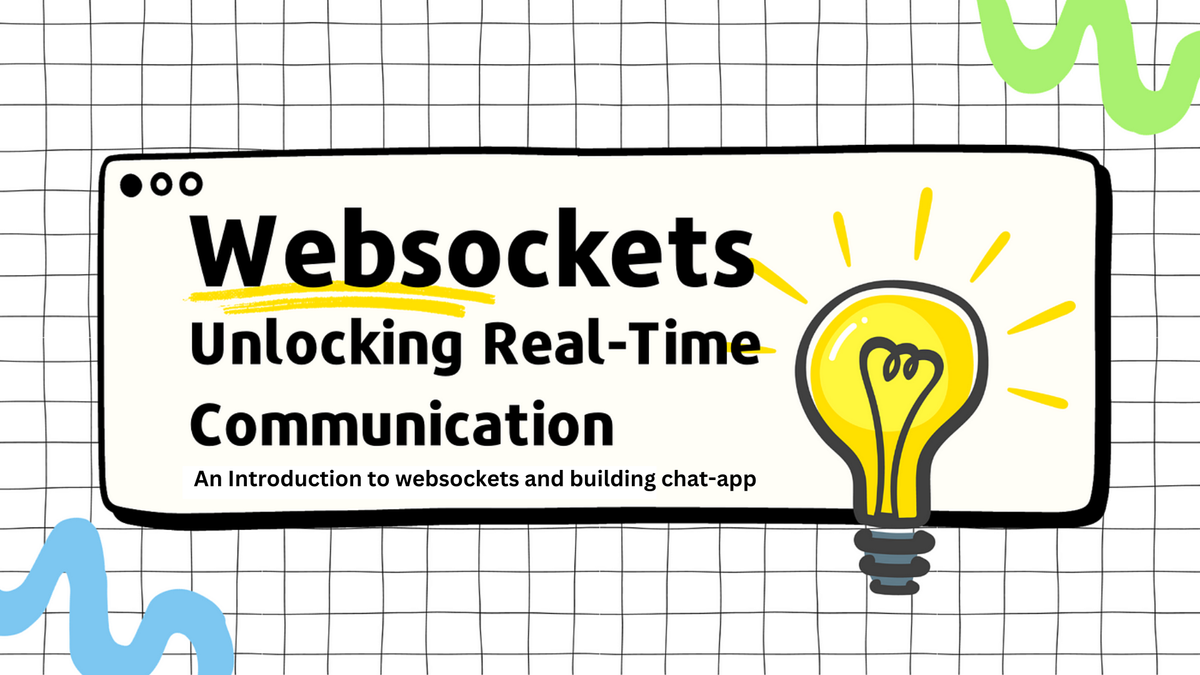
In today's data-driven world, real-time dashboards are essential for monitoring critical systems and making informed decisions. WebSockets have emerged as a powerful technology for enabling real-time data updates in web applications. This blog post will delve into the world of real-time dashboards, exploring WebSockets in both Angular and React. We'll then compare and contrast their implementation approaches, highlighting the strengths and considerations for each framework. Finally, we'll go beyond WebSockets, discussing cutting-edge technologies like Server-Sent Events (SSE) and GraphQL subscriptions for even more dynamic experiences. Throughout, we'll provide code examples and performance benchmarks to illustrate the concepts.
The Power of WebSockets
WebSockets are a communication protocol that provides a persistent, two-way connection between a web client and a server. This bi-directional nature is what sets WebSockets apart from traditional HTTP requests, which are one-way and short-lived. With WebSockets, the server can pro-actively push data to the client, enabling real-time updates without requiring the client to constantly poll the server. This significantly reduces latency and improves the responsiveness of your dashboard.
WebSockets in Angular
Angular provides a straightforward way to leverage WebSockets using the WebSocket
class. Here's a code example demonstrating a basic WebSocket
import { Injectable } from '@angular/core';
import { Observable, webSocket } from 'rxjs';
import { map } from 'rxjs/operators';
@Injectable({ providedIn: 'root' })
export class WebSocketService {
constructor() {}
createWebSocketConnection(url: string): Observable<any> {
return webSocket(url).pipe(
map((message: any) => JSON.parse(message))
);
}
}
This service creates a WebSocket observable and parses incoming JSON messages. You can inject this service into your components and subscribe to the observable to receive real-time data updates.
WebSockets in React
React adopts a more library-based approach for WebSockets. Popular libraries like socket.io-client
or ws
provide functionalities for creating and managing WebSocket connections. Here's an example using socket.io-client
:
import io from 'socket.io-client';
const socket = io('http://localhost:3000');
socket.on('message', (data) => {
console.log('Received data from server:', data);
// Update your UI components here
});
// Send data to the server
socket.emit('sendData', { message: 'Hello from React!' });
This example creates a Socket.IO connection and listens for the 'message' event to receive data from the server. You can similarly emit events from the client to send data to the server.
Comparison: Angular vs. React
Both Angular and React offer effective solutions for building real-time dashboards with WebSockets. Angular's WebSocket
class provides a more integrated approach, while React leverages third-party libraries for flexibility. Here's a table summarizing the key considerations:
Feature | Angular | React |
---|---|---|
WebSocket Implementation | Built-in WebSocket class | Third-party libraries (e.g., socket.io-client) |
Development Style | More structured and opinionated | More flexible and component-based |
Data Binding | Two-way data binding | One-way data flow (state management required) |
Learning Curve | Steeper due to framework complexity | Easier to learn for beginners |
The best choice between Angular and React depends on your project requirements and team preferences. If you value a structured approach with built-in features, Angular might be a good fit. If you prefer flexibility and a component-based architecture, React could be the way to go.
Beyond WebSockets: SSE and GraphQL Subscriptions
While WebSockets are a powerful tool, they are not the only option for real-time data updates. Here are two other technologies to consider:
Server-Sent Events (SSE):
SSE is a simpler protocol compared to WebSockets. It allows the server to push data to the client without a persistent connection. This can be a good choice for scenarios where real-time updates are less frequent or where browser compatibility is a concern.
GraphQL Subscriptions:
GraphQL is a query language for APIs. GraphQL subscriptions enable clients to subscribe to specific events or data changes within a GraphQL schema. This approach offers a more structured way to manage real-time data updates within a GraphQL ecosystem
Conclusion
Building real-time dashboards has become an essential capability for modern web applications. WebSockets provide a powerful mechanism for enabling bi-directional communication and real-time data updates. This blog post explored WebSockets in the context of Angular and React, highlighting their implementation approaches and key considerations. We also ventured beyond WebSockets, discussing Server-Sent Events (SSE) and GraphQL Subscriptions for even more dynamic experiences.
Choosing the right technology for your real-time dashboard depends on your specific needs. WebSockets offer the lowest latency and greatest flexibility, but require more complex implementation. SSE can be a simpler alternative for less frequent updates. GraphQL subscriptions provide a structured approach within a GraphQL ecosystem.
By carefully considering these factors and leveraging the code examples provided, you can build blazing-fast real-time dashboards that keep your users informed and in control. Remember, the choice of framework (Angular vs React) can also influence your implementation approach.
In addition to the technologies discussed here, other options like WebRTC and long polling can also be explored for real-time communication. The key is to identify the approach that best aligns with your project requirements and development expertise.
I hope this blog post has been informative. Feel free to leave comments or questions below, and happy building!