Architecting Scalable and Maintainable UI Components in Angular and React
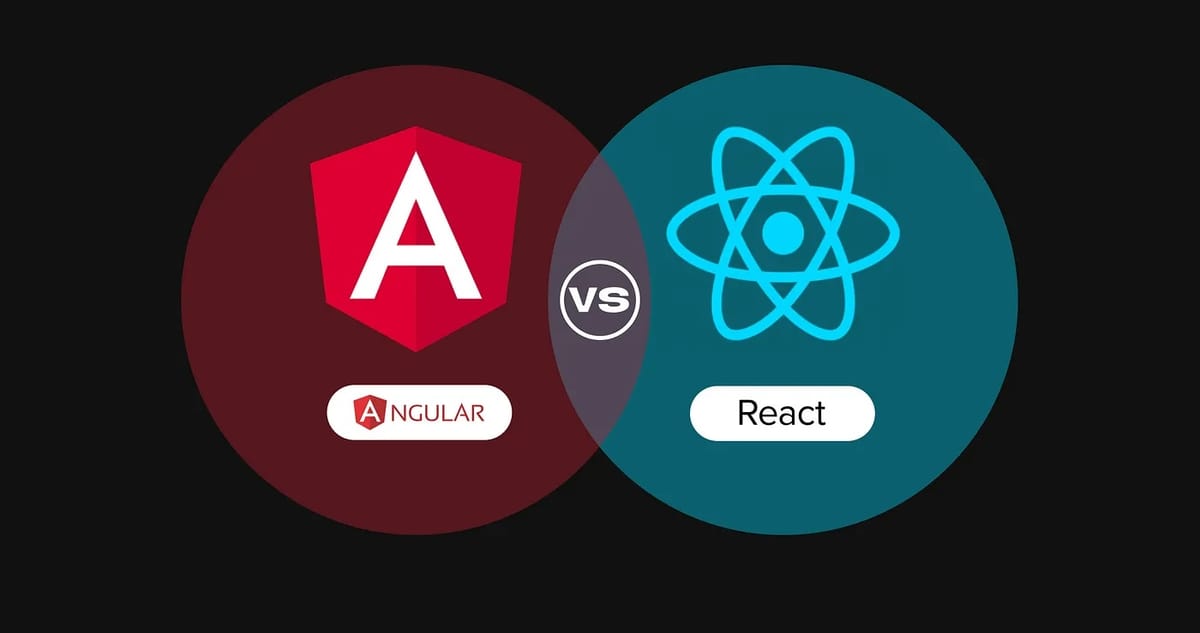
In the realm of modern web development, creating scalable and maintainable UI components is crucial for building robust and efficient applications. Angular and React, two of the most popular JavaScript frameworks, offer powerful tools and methodologies for crafting reusable and maintainable UI components. In this article, we'll delve into the best practices and strategies for building scalable UI components in both Angular and React, covering various aspects including component architecture, composition, props vs. inputs, directives, lifecycle methods, and component testing.
Component Architecture:
Both Angular and React follow component-based architectures, where the UI is composed of reusable and self-contained components. In Angular, components are the fundamental building blocks, encapsulating HTML templates, CSS styles, and TypeScript logic. React, on the other hand, utilizes functional or class-based components to define UI elements.
Angular Component Architecture:
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
// Component logic goes here
}
React Component Architecture:
import React from 'react';
const ExampleComponent = () => {
// Component logic goes here
return (
<div>
{/* JSX for rendering UI */}
</div>
);
};
export default ExampleComponent;
Component Composition:
Both Angular and React encourage component composition, allowing developers to build complex UIs by combining smaller, reusable components. This promotes code reusability and simplifies maintenance.
Props vs. Inputs:
In React, components receive data through props, which are read-only. In Angular, inputs serve a similar purpose but offer additional features like type checking and change detection.
React Props:
const ParentComponent = () => {
return <ChildComponent name="John" />;
};
const ChildComponent = (props) => {
return <div>Hello, {props.name}!</div>;
};
Angular Inputs:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: '<div>Hello, {{ name }}!</div>'
})
export class ChildComponent {
@Input() name: string;
}
Directives:
Angular and React utilize directives for manipulating the DOM and adding behavior to components. Angular provides built-in directives like ngIf, ngFor, and ngClass, while React relies on custom or third-party libraries for similar functionality.
Lifecycle Methods:
Both frameworks offer lifecycle methods for managing component initialization, rendering, and destruction. Understanding these lifecycle hooks is essential for optimizing performance and handling side effects.
Component Testing:
Testing UI components is integral to ensuring their reliability and stability. Angular and React provide testing frameworks (Jasmine/Karma for Angular, Jest for React) along with utilities for writing unit tests and performing component-level testing.
In conclusion, building scalable and maintainable UI components in Angular and React requires a deep understanding of each framework's principles and best practices. By following component-based architectures, embracing composition, leveraging props/inputs effectively, utilizing directives, mastering lifecycle methods, and implementing comprehensive testing strategies, developers can create robust and efficient UIs that scale with their applications' needs.
Whether you're working with Angular or React, prioritizing clean and maintainable code will ultimately lead to better user experiences and smoother development workflows. With the right approach and mindset, crafting UI components that stand the test of time becomes not only achievable but also a rewarding endeavor in the journey of web development.